jQuery:1.基础
jQuery是一个封装好的js库,目的是让你‘写得更少,做得更多’。
1.jQuery选择器
基础选择器
几个容易忘记的选择器
1 2 3 4
| $('div,p,li') $('li.current') $('ul>li') $('ul li')
|
筛选选择器
1 2 3 4 5
| $('li:first') $('li:last') $('li:eq(2)') $('li:odd') $('li:even')
|
筛选方法(重点)
1 2 3 4 5 6 7 8
| $('li').parent() $('ul').children('li') $('ul').find('li') $('.first').siblings('li') $('.first').nextAll() $('.last').preAll() $('div').hasClass('protected') $('li').eq(2)
|
排他思想实现
1 2 3 4
| <button>Button</button> <button>Button</button> <button>Button</button>
|
1 2 3 4 5 6 7
| <script> $('button').click(function () { $(this).css('background-color','pink'); $(this).siblings('button').css('background-color',''); }); </script>
|
tips: jquery自带隐式迭代
1.淘宝购物服饰精选(排他思想实现)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
|
<div class="box"> <div class="left"> <ul> <li>0</li> <li>9</li> </ul> </div> <div class="right"> <div>0</div> <div>9</div> </div> </div>
<script> $(function () { $('.right>div').eq(0).show(); $('.left ul li').click(function () { $(this).css('background-color','yellowgreen'); $(this).siblings().css('background-color',''); var index = $(this).index(); $('.right>div').eq(index).show(); $('.right>div').eq(index).siblings('div').hide(); }) }) </script>
<script> $('.right>div').eq(index).show().siblings('div').hide(); </script>
|
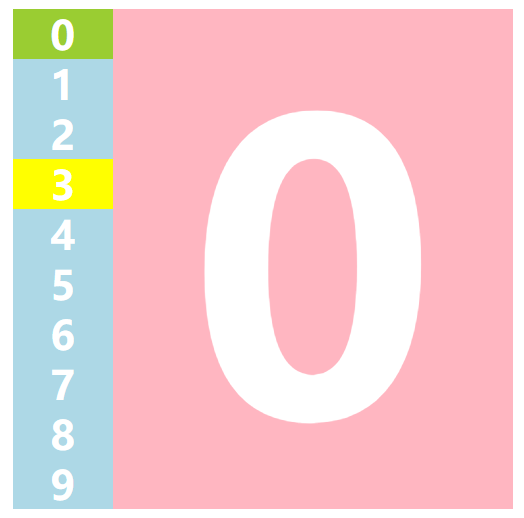
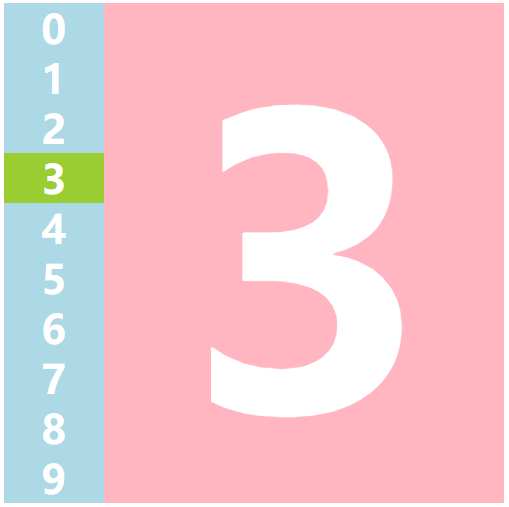
2.jQuery样式修改
jq的css表达式只有一个参数时,获取该参数的值
1 2 3 4
| $('选择器').css('样式名');
console.log($('div').css('width'));
|
jq的css表达式有两个参数时,修改第一个参数css的值为第二个参数
1 2 3 4 5 6 7 8
| $('选择器').css('样式名',‘值’)
$('div').css('width',‘300px’)
var div = document.querySelector('div'); div.style.width = '300px';
$('div').css('width',300);
|
一次性修改多项参数的时候,可以使用对象的写法
1 2 3 4 5 6 7 8
| $('div').css({ 'width': '400px', height: 400, backgroundColor: 'black' })
|
类操作(实例请查看购物车修改商品背景部分)
1 2 3
| $('选择器').addClass('css类名'); $('选择器').removeClass('css类名'); $('选择器').toggleClass('css类名');
|
tips: 类名不要加点’.’,Class记得C大写
3.jQuery效果API
下滑上滑(下拉菜单实例)
1 2 3
| $('选择器').slideDown(500,swing,fn); $('选择器').slideUp(500,swing,fn); $('选择器').slideToggle(500,swing,fn);
|
淡入淡出(图片聚焦实例)
1 2 3 4
| fadeIn([s],[e],[fn]) fadeOut([s],[e],[fn]) fadeTo([[s],o,[e],[fn]]) fadeToggle([s,[e],[fn]])
|
自定义动画
1
| animate(params,[speed],[easing],[fn])
|
tips:params需要以对象的形式传入
1.下拉菜单更加简便的写法(hover())
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
|
<script> $(function () { $('body>ul>li').mouseover(function () { $(this).children('ul').show(); }); $('body>ul>li').mouseleave(function () { $(this).children('ul').hide(); }); }); </script>
<script> $(function () { $('body>ul>li').hover(function () { $(this).children('ul').slideDown(); },function f() { $(this).children('ul').slideUp(); }) }); </script>
<script> $(function () { $('body>ul>li').hover(function () { $(this).children('ul').slideToggle(); }) }); </script>
<script> $(function () { $('body>ul>li').hover(function () { $(this).children('ul').stop().slideToggle(); }) }); </script>
|
2.图片焦点聚集实例
原理:将选中的图片的兄弟透明度变为0.5
1 2 3 4 5 6 7 8 9 10
| <!--css和html略--> <script> $(function () { $('.box div').hover(function () { $(this).siblings('div').stop().fadeTo(500,0.5) },function () { $(this).siblings('div').stop().fadeTo(500,1); }) }) </script>
|
效果图
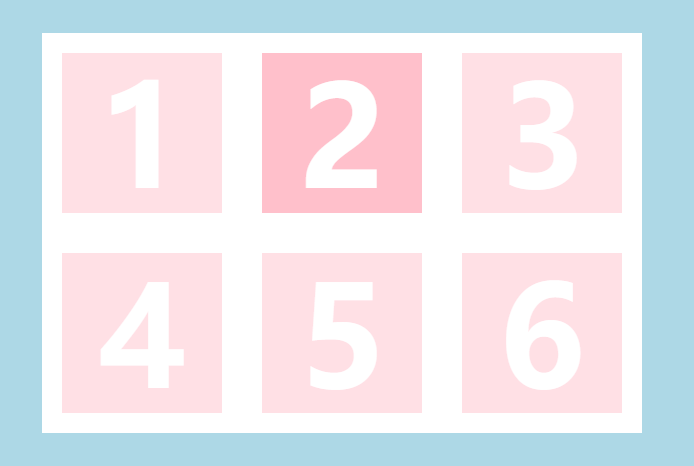
3.手风琴效果简单实现



1 2 3 4 5 6 7 8 9 10 11 12
| <ul> <li class="odd"> <div class="big odd">这个是1</div> <div class="small odd">1</div> </li> <li class="even"> <div class="big odd">这个是8</div> <div class="small odd">8</div> </li> </ul>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| li>div{ color: #11999e; font-weight: bolder; font-size: 40px; line-height: 60px; } ul { width: 620px; height: 60px; margin: 200px auto; } li { float: left; width: 60px; height: 60px; } .big { display: none; height: 60px; width: 200px; text-align: center; } .small { display: inline-block; height: 60px; width: 60px; text-align: center; } .odd .big { background-color: #cbf1f5; } .odd .small{ background-color: #e3fdfd; } .even .big { background-color: #a6e3e9; } .even .small{ background-color: #71c9ce; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| $(function () { $('li').eq(0).css('width','200px'); $('li').eq(0).children('.big').show(); $('li').eq(0).children('.small').hide();
$('li').mouseover(function () { $(this).siblings('li').stop().animate({'width':'60px',},500); $(this).stop().animate({'width':'200px',},500); $(this).children('.big').stop().fadeIn('fast').siblings('.small').stop().fadeOut('fast'); $(this).siblings('li').children('.big').stop().fadeOut('fast').siblings('.small').stop().fadeIn('fast'); }) })
|
4.jQuery获取属性
获取元素固有属性
1 2 3 4
| $('选择器').prop('属性');
$('选择器').prop('属性','值');
|
获取元素的自定义属性
1 2 3 4
| $('选择器').attr('属性');
$('选择器').attr('属性','值');
|
5.jQuery获取文本值
设置获取元素内容
1 2 3 4
| $('选择器').html();
$('选择器').html('内容');
|
设置获取元素文本内容
1 2 3 4
| $('选择器').text();
$('选择器').text('内容');
|
获取设置表单值
1 2 3 4
| $('选择器').val();
$('选择器').val('内容');
|
购物车功能
详情查看P058购物车案例
6.jQuery遍历元素
1 2 3 4 5 6
| $(element).each(function(index,element){ })
|
7.jQuery创建元素
1
| var li = $('<li></li>');
|
8.jQuery添加与删除元素
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| $('ul').append('<li></li>');
$('ul').prepend('<li></li>'first);
$('.first').after('<div class='second'></div>');
$('.second').after('<div class='first'></div>');
$('element').remove();
$('element').empty(); $('element').html('');
|
jQuery:2.事件操作
事件绑定
1
| $('ele').on(events[,select],fn);
|
实例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| $('div').on('mouseenter',function(){ $(this).css('background-color','skyblue'); })
$('div').on({ mouseenter:function () { $(this).css('background-color','#95e1d3'); }, click:function () { $(this).css('background-color','#eaffd0'); }, mouseleave:function () { $(this).css('background-color','#fce38a'); } })
$('div').on('mouseenter mouseleave',function () { $(this).toggleClass('current'); })
|
事件委派
1 2 3 4 5
| $('ele').on(events[,select],fn);
$('ul').on('click','li',function(){ })
|
tips:这里on可以给未来的元素绑定事件
事件解绑
1 2 3
| $('ele').off() $('ele').off('click') $('ele').off('click','sonEle')
|
tips:如果想让绑定的事件只执行一次,可以使用one()**取代on()**
自动触发事件
1 2 3
| $('ele').click() $('ele').trigger('click') $('ele').triggerHandler('click')
|
事件对象
阻止冒泡
1 2 3 4 5 6 7 8 9 10 11 12 13
| <div class="parent"> <div class="son"></div> </div> <script> $('.parent').on('click',function(){ console.log('父盒子'); }) $('.son').on('click',function(event){ console.log('子盒子'); event.stopPropagation(); }) </script>
|
jQuery:3.对象操作
1
| $.extend( [deep ], target, object1 [, objectN ] )
|
tips:注意深浅拷贝的概念
- 浅拷贝:对于简单数据来说,深拷贝和浅拷贝都一样,会创建一个新的值。但是对于复杂数据如对象,会直接引用合并前的对象内容,所以修改新对象中的数据会影响到原对象的数据。
- 深拷贝:会对对象中的每个数据都进行复制,遇到复杂数据也会重新创建一个一模一样的对象,而不是引用。
jQuery:4.位置操作
**offset()**:获取设置文档的偏移值
1 2 3 4 5 6
| $(ele).offset(); $(ele).offset().top; $(ele).offset({ top:200, left:200 });
|
注意设置的时候要以对象的形式